Filament Repeater Field Unique Validation

OsamaNagi
@OsamaNagi
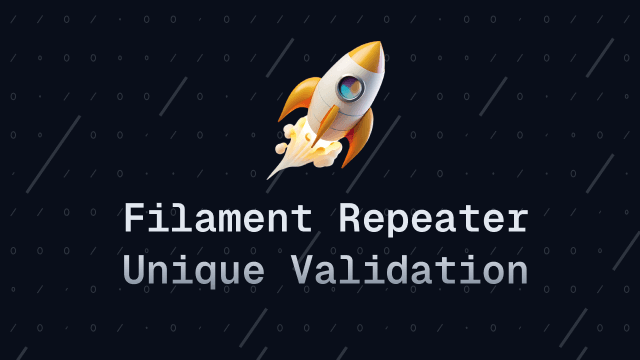
Filament's repeater field is a powerful tool for creating multiple entries of the same type. However, ensuring that each entry is unique can be a challenge. In this guide, we'll walk through how to implement unique validation for repeater fields in Filament using a Laravel
custom rule.
Introduction
When working with repeater fields, you may want to ensure that certain fields within the repeater are unique across all entries. For example, if you're creating a list of users, you might want to ensure that each user has a unique email address. This is where custom validation rules come into play. By creating a custom rule, you can enforce unique constraints on the fields within the repeater.
Example Scenario
Let's say we have a repeater field for adding multiple options to a form, and we want to ensure that each option has a unique name. We'll create a custom validation rule that checks for uniqueness within the repeater field.
Forms\Components\Repeater::make('options')
->schema([
Forms\Components\TextInput::make('option')
->required()
->dehydrateStateUsing(function ($state) {
return Str::upper($state);
})
->maxLength(255)
])
->reorderableWithButtons()
->required(),
In this example, we have a repeater field named options
with a text input for each option. We want to ensure that the option
field is unique across all entries in the repeater.
To achieve this, we'll create a custom validation rule that checks for uniqueness within the repeater field.
Solution
To implement unique validation for repeater fields in Filament, we can leverage Laravel's custom validation rules. Here's how to do it:
->rule(fn () => function (string $attribute, $value, Closure $fail) {
$optionValues = collect($value)->pluck('option');
$duplicates = $optionValues->duplicates();
if ($duplicates->isNotEmpty()) {
$fail('The options must be unique.');
}
})
In this code snippet, we define a custom validation rule that checks for duplicate values in the option
field of the repeater. If any duplicates are found, we use the $fail
callback to return an error message.
! NOTE This validation rule should be added to the
Repeater
field it self, not the individual fields within the repeater. This ensures that the validation checks all entries in the repeater.
Complete Example
Here's the complete example of how to implement unique validation for a repeater field in Filament:
Forms\Components\Repeater::make('options')
->schema([
Forms\Components\TextInput::make('option')
->required()
->dehydrateStateUsing(function ($state) {
return Str::upper($state);
})
->maxLength(255)
])
->rule(fn () => function (string $attribute, $value, Closure $fail) {
$optionValues = collect($value)->pluck('option');
$duplicates = $optionValues->duplicates();
if ($duplicates->isNotEmpty()) {
$fail('The options must be unique.');
}
})
->reorderableWithButtons()
->required(),
That's it! Now, when you try to submit the form with duplicate options in the repeater field, you'll receive a validation error message indicating that the options must be unique.
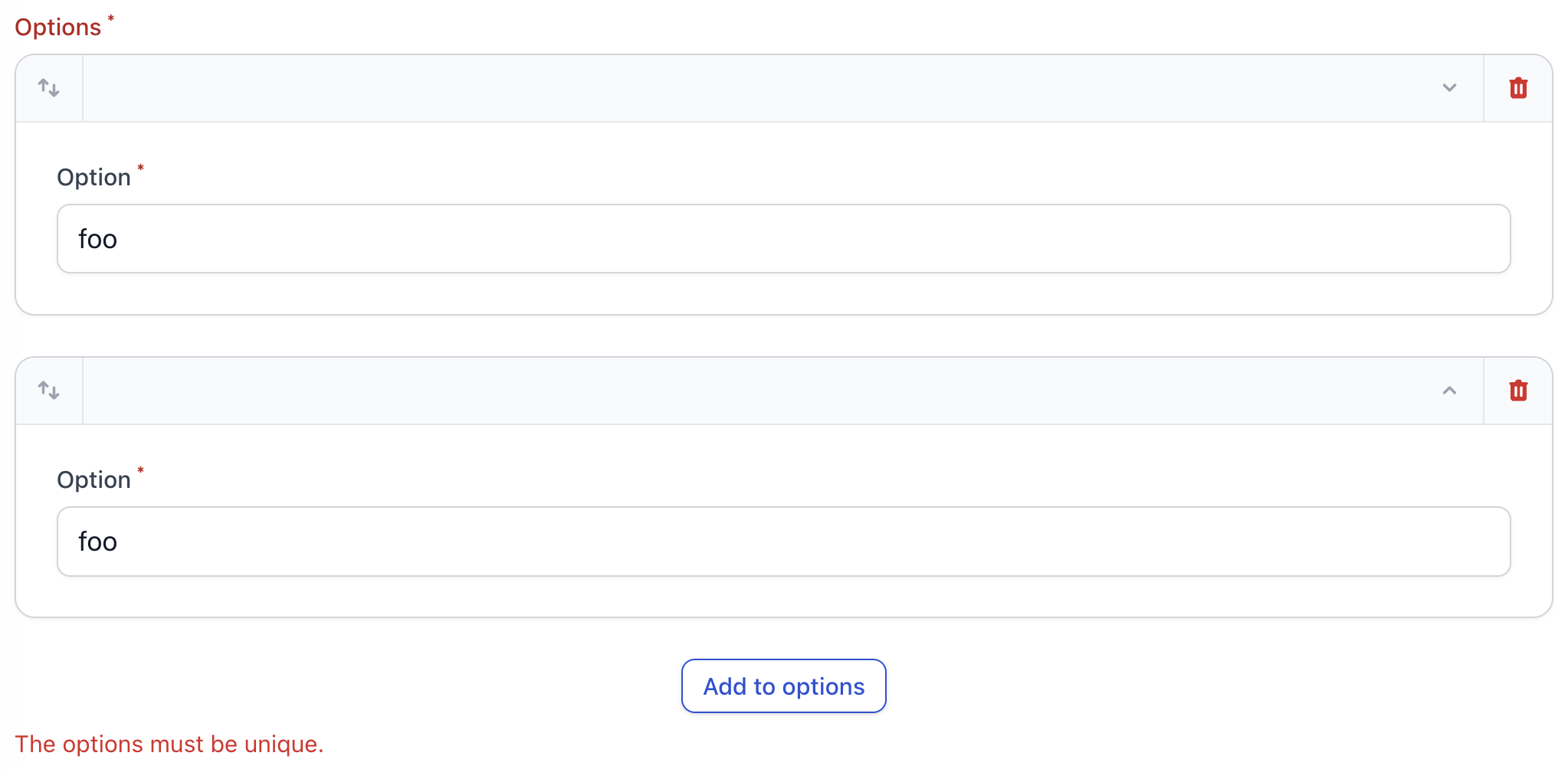